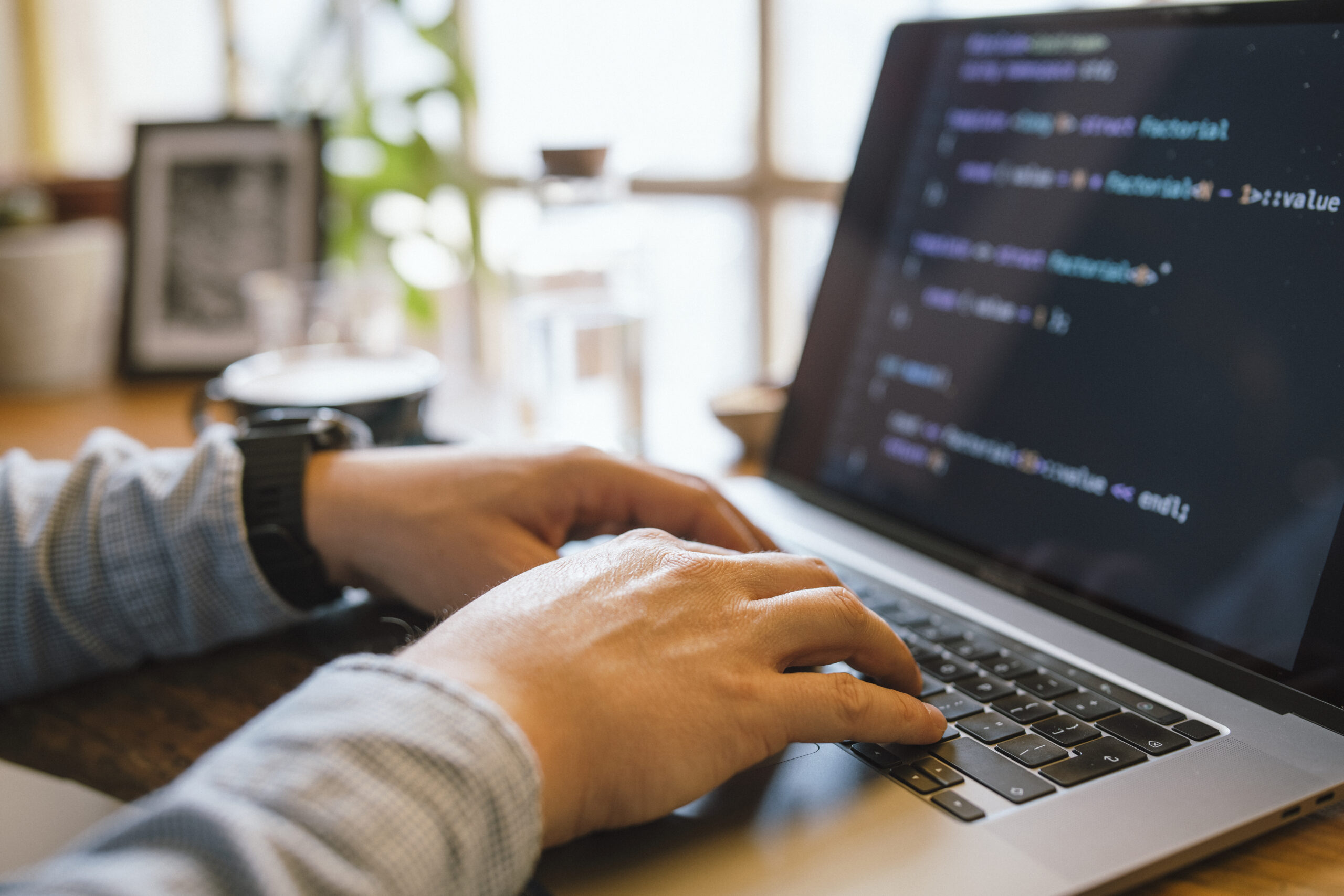
Debugging is One of the more important — nevertheless normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of annoyance and considerably transform your productiveness. Allow me to share many approaches to aid developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although writing code is one Element of progress, being aware of the best way to interact with it proficiently for the duration of execution is equally important. Modern-day growth environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the floor of what these resources can perform.
Just take, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources let you set breakpoints, inspect the value of variables at runtime, move by way of code line by line, and in some cases modify code about the fly. When utilized correctly, they Enable you to observe particularly how your code behaves for the duration of execution, which is priceless for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-stop developers. They permit you to inspect the DOM, observe network requests, watch genuine-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can change disheartening UI concerns into workable tasks.
For backend or technique-amount developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle about running processes and memory administration. Discovering these resources could have a steeper Discovering curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Command systems like Git to comprehend code heritage, obtain the precise moment bugs were introduced, and isolate problematic adjustments.
In the long run, mastering your instruments usually means going beyond default settings and shortcuts — it’s about building an intimate understanding of your enhancement ecosystem to ensure that when problems come up, you’re not misplaced at nighttime. The higher you recognize your equipment, the more time it is possible to commit fixing the actual issue instead of fumbling via the procedure.
Reproduce the condition
One of the more significant — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Just before jumping into the code or earning guesses, builders need to have to create a consistent ecosystem or circumstance the place the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing a problem is accumulating as much context as possible. Talk to inquiries like: What actions triggered the issue? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact disorders beneath which the bug occurs.
As soon as you’ve collected ample info, endeavor to recreate the trouble in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic checks that replicate the edge scenarios or state transitions concerned. These assessments not only support expose the issue and also prevent regressions Later on.
In some cases, the issue could be natural environment-specific — it would transpire only on certain working programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible state of affairs, You may use your debugging tools more successfully, check prospective fixes securely, and talk a lot more Obviously along with your group or consumers. It turns an abstract complaint right into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to take care of error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it took place, and occasionally even why it happened — if you know how to interpret them.
Get started by looking through the message carefully As well as in total. Many builders, particularly when under time force, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them very first.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line variety? What module or function induced it? These thoughts can information your investigation and issue you toward the dependable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, As well as in These scenarios, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings either. These frequently precede more substantial challenges and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach commences with being aware of what to log and at what degree. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of growth, Information for common occasions (like successful get started-ups), Alert for likely concerns that don’t break the applying, Mistake for real issues, and Lethal if the program can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, condition changes, enter/output values, and demanding conclusion factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, wise logging is about stability and clarity. That has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, gain deeper visibility into your apps, and Increase the overall maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it is a form of investigation. To efficiently establish and take care of bugs, developers should technique the procedure similar to a detective resolving a mystery. This state of mind aids stop working advanced challenges into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant information as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What might be creating this behavior? Have any changes a short while ago been built to your codebase? Has this situation transpired prior to under identical situation? The purpose is always to narrow down possibilities and establish likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, request your code questions and Permit the outcomes guide you nearer to the reality.
Pay back near interest to compact information. Bugs frequently disguise inside the the very least predicted places—just like a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty with out thoroughly knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Last of all, maintain notes on That which you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for long term troubles and assistance Other individuals fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate methods.
Publish Assessments
Crafting tests is one of the best strategies to transform your debugging skills and General advancement effectiveness. Assessments don't just help catch bugs early but also serve as a safety net that gives you self-assurance when generating improvements towards your codebase. A perfectly-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with unit tests, which focus on individual functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know in which to appear, considerably reducing some time expended debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier getting set.
Next, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance make sure a variety of elements of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex devices with several components or expert services interacting. If one thing breaks, your checks can inform you which part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to Imagine critically about your code. To check a characteristic properly, you require to know its inputs, envisioned outputs, and edge instances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to focus on fixing the bug and look at your exam pass when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you capture additional bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the trouble—observing your display screen for several hours, seeking solution right after Remedy. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new perspective.
When you're too near the code for too long, cognitive exhaustion sets in. You might start overlooking obvious faults or misreading code that you choose to wrote just several hours before. With this condition, your brain turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your read more concentrate. Many builders report obtaining the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, having breaks isn't a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something useful in case you make the effort to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code evaluations, or logging? The answers usually reveal blind spots inside your workflow or comprehending and assist you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see styles—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as essential portions of your improvement journey. In fact, several of the best builders are not the ones who generate excellent code, but those who continually learn from their problems.
In the end, Just about every bug you repair provides a new layer in your talent set. So following time you squash a bug, have a moment to mirror—you’ll appear away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills will take time, exercise, and patience — nevertheless the payoff is big. It will make you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at That which you do.